4 libraries to use in your React app
React is a view library - the V in MVC. To be able to write a modern and complex React applications we need to include more libraries than only React.
But which ones should you use? In a quickly changing landscape, it is easy to miss out on essential libraries.
In this article, I list the 4 libraries you should know about and consider for your next React application.
1. Redux
When your React application grows, you will soon notice that you send many callbacks around for managing the state that is shared between React components. At this point, it can be a bit difficult to follow the flow of what is happening.
If you are in this situation then it’s time to look into a library for managing state. The most widely used one in React ecosystem is called Redux.
With Redux you have one store for the whole application state. Redux makes it easy and reliable for any component to access and update the state in the store.
Learning resources
2. GraphQL
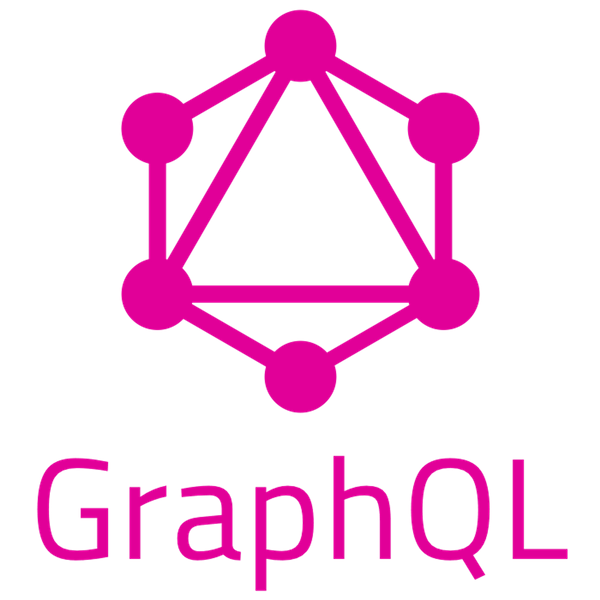
GraphQL is used for fetching data to your components from a backend instead of using REST API calls.
Advantages compared to REST:
-
You define in the front end exactly what kind of data to use for each component.
-
Only the data needed for the components are sent over the network.
-
You don’t need to write many API endpoints, you only have one
/graphql
endpoint. -
It has powerful developer tools that helps you visualize the data.
Disadvantages:
-
There is a learning curve.
-
Not as widely used as REST by existing public and private APIs.
On the backend, you can create a GraphQL endpoint for your data with graphql.js together with a library for the web server you are using for example express-graphql. You can also use a SaaS for example graph.cool
On the frontend, you can use a library for connecting GraphQL to your react components. The two biggest ones right now are Appollo and Relay.
Learning resources
3. Lodash
Lodash is a library with JavaScript utility functions mainly used for manipulating data structures. ES6 already has many of these functions implemented, but lodash still have some advantages compared to using pure ES6.
Advantages with lodash compared to ES6:
-
Better performance
-
Consistent API. Compare lodash
_.keys()
and_.map()
to ES6Object.keys()
and[23, 1].map()
-
You can elegantly chain multiple calls which make the code more readable
-
null safety on all calls. In ES6 you will get a runtime error if you do
data.map()
anddata
isundefined
. This will not happen with lodash. -
Usable utility functions which ES6 lacks, for example,
_.get
,_.flatten
,_.clone
and_.groupby
Disadvantages:
-
It’s another dependency in your project which adds to your bundle size
-
It’s another new thing for you and your team mates to learn.
Learning resources
-
Experiment with it! :)
4. Recompose
Recompose is a library that provides a toolkit of helper functions for working with functional components and Higher Order-Components.
Recompose has many features. I want to highlight these features:
-
Access lifecycle methods in functional components
-
Add state to functional components
-
Compose HOCs.
With recompose, you can code your React application entirely with functional components.
Recompose is a library for advanced users. I recommend using it only if you are comfortable working with functional components and/or HOCs in your code base.