Create a React app with zero configuration using Parcel
We have all experienced the pain of getting started with React. You spend hours to configure webpack before you can start actual coding.
Create React App was created to make it easier and quicker to get started. The problem with create react app is that it hides the webpack config. When your app grows and you need something a bit more advanced, you have to eject and then you get a huge webpack config. And then you are back to the problem that you need to learn webpack anyway.
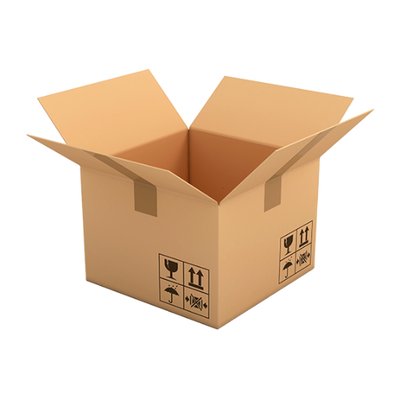
I tried it on a large code base, and it worked out-of-the-box! It even gave me a bundle that was optimized. I have spent days optimizing that bundle using webpack.
I think this tool has potential. Let’s look at how to create a React app from scratch!
Create a React app with Parcel
First, we create a new NPM app:
mkdir react-parcel
cd react-parcel
npm init
npm init will ask you a bunch of questions. Just press enter for the defaults.
next add the dependencies for React, Babel and Parcel:
npm install --save react
npm install --save react-dom
npm install --save-dev @babel/preset-react
npm install --save-dev @babel/preset-env
npm install --save-dev parcel-bundler
Babel needs two dependencies, one for supporting JSX, and also @babel/preset-env.
Next, we create the .babelrc file. This file tells parcel that we are using ES6 and React JSX!
{
"presets": ["@babel/preset-react"]
}
Next create our react app. It’s only two files. First index.js
:
import React from "react"
import ReactDOM from "react-dom"
class HelloMessage extends React.Component {
render() {
return <div>Hello {this.props.name}</div>
}
}
var mountNode = document.getElementById("app")
ReactDOM.render(<HelloMessage name="Jane" />, mountNode)
…and then index.html
:
<!DOCTYPE html>
<html>
<head>
<title>React starter app</title>
</head>
<body>
<div id="app"></div>
<script src="index.js"></script>
</body>
</html>
Now we just need to add a script entry to package.json to be able to start our app
"scripts": {
"start": "parcel index.html",
},
We are done. Let’s start our app:
npm start
Currently you must use node 8 (or later). Now browse to http://localhost:1234 to see the app!
Thoughts
Compare this to the config you have to write if you are using webpack. This was a lot simpler!
Parcel looks like a decent alternative to Create React App. Is it ready for production on large application? Not sure, we have to see how things develop. It is going to be interesting to follow!
Follow me on Twitter to get real-time updates with tips, insights, and things I build in the frontend ecosystem.